Project Details
Random Password Generator
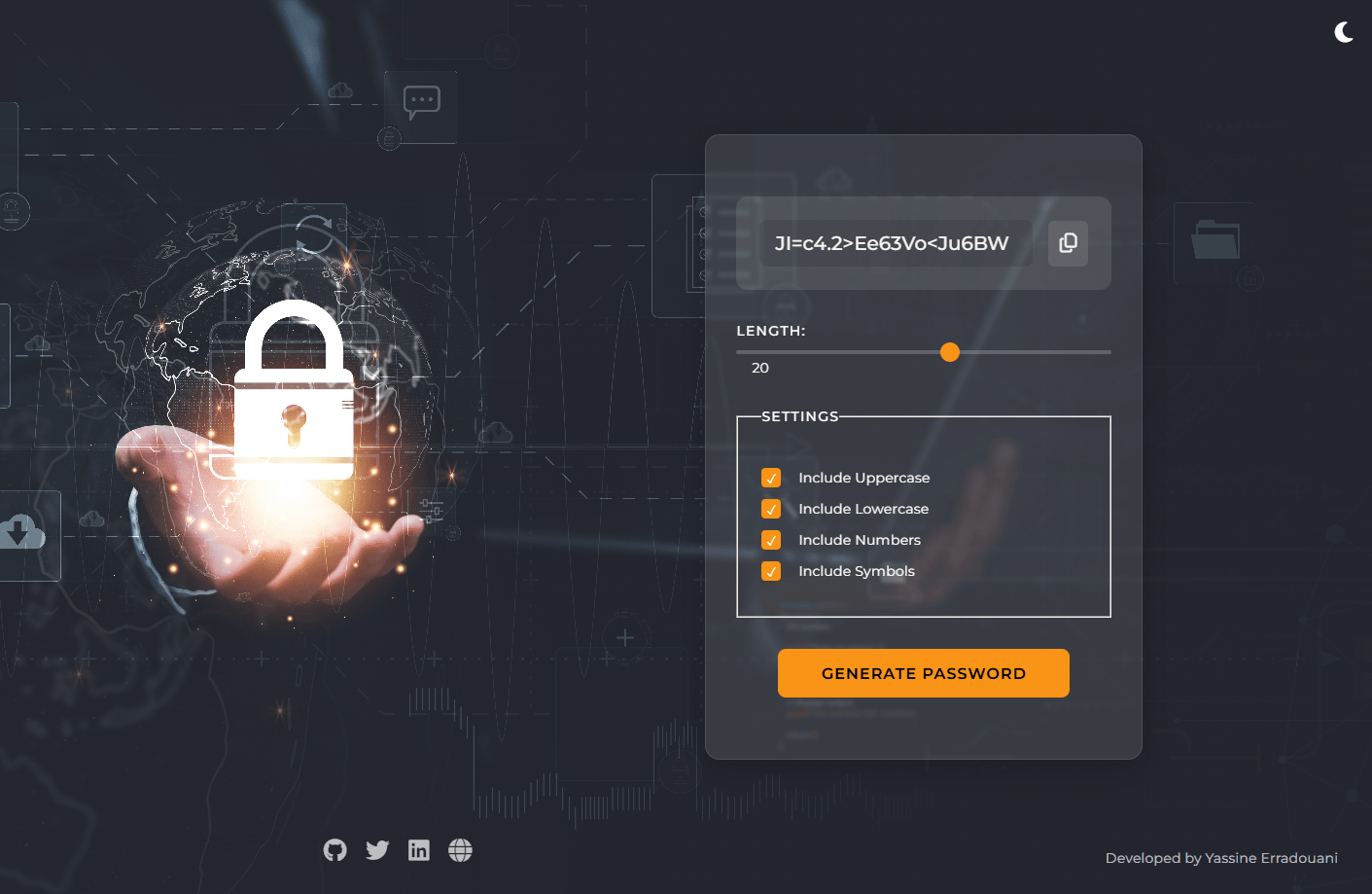
Main interface of the Password Generator
Technologies Used
Project Overview
YPass Password Generator is a versatile and secure tool designed to help users create strong, random passwords effortlessly. Whether you're looking to enhance your online security, manage multiple accounts, or simply generate complex passwords for various applications, YPass offers a user-friendly interface and robust features to meet your needs.
Key Features
- Customizable Password Length: Specify the exact length of the password to suit different security requirements.
- Character Set Selection: Choose to include or exclude uppercase letters, lowercase letters, numbers, and special characters.
- User-Friendly Interface: Intuitive design for easy navigation and usage, suitable for both beginners and advanced users.
- Clipboard Integration: Copy generated passwords directly to the clipboard with a single click.
- Randomness Assurance: Utilizes secure randomization algorithms to ensure unpredictability.
Code Snippet
Javascript
document.getElementById('generate').addEventListener('click', () => { const length = document.getElementById('slider').value; const result = document.getElementById('result'); // Password generation logic const charset = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789!@#$%^&*()_+"; let password = ""; for (let i = 0; i < length; i++) { const randomIndex = Math.floor(Math.random() * charset.length); password += charset[randomIndex]; } result.textContent = password; }); document.getElementById('generate').addEventListener('click', generatePassword); function generatePassword() { // Get selected options const uppercase = document.getElementById('uppercase').checked; const lowercase = document.getElementById('lowercase').checked; const numbers = document.getElementById('numbers').checked; const symbols = document.getElementById('symbols').checked; const length = document.getElementById('slider').value; // Define character sets const uppercaseChars = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'; const lowercaseChars = 'abcdefghijklmnopqrstuvwxyz'; const numberChars = '0123456789'; const symbolChars = '!@#$%^&*()_+-=[]{}|;:,.<>?'; // Build character set based on selected options let chars = ''; if (uppercase) chars += uppercaseChars; if (lowercase) chars += lowercaseChars; if (numbers) chars += numberChars; if (symbols) chars += symbolChars; // Generate password let password = ''; if (chars === '') { password = 'Please select at least one option'; } else { for (let i = 0; i < length; i++) { const randomIndex = Math.floor(Math.random() * chars.length); password += chars[randomIndex]; } } // Display password document.getElementById('result').textContent = password; } document.getElementById('copy-btn').addEventListener('click', async () => { const password = document.getElementById('result').textContent; try { await navigator.clipboard.writeText(password); document.querySelector('.result__info.left').style.opacity = '1'; setTimeout(() => { document.querySelector('.result__info.left').style.opacity = '0'; }, 2000); } catch (err) { console.error('Failed to copy password:', err); } });